What is an Object?
- An object can be considered a "thing" that can perform a set of related activities.
- Any real entity.
For example:
- The hand can grip something.
- A fish in water (fish is an object).
- An idea comes in your mind, and you implement it (the idea is an object).
- Fruit in an orchard (fruit is an object).
What is a Class?
A class is simply a representation of a type of object. It is the blueprint / plan / template that describes the details of an object. A class is the blueprint from which the individual objects are created.
For example:
Take an example of a bowl in which you can bake a cake, the cake could be of different flavors so cake is my class and its flavors are objects.

CODE:
How can you create a class and object in C#?
class Program{
static void Main(string[] args)
{
baby aisha = new baby();
aisha.crawl();
Console.WriteLine("i am too little so i can crawl only :-)");
Console.ReadLine();
aisha.food();
Console.WriteLine("i eat mashed potatoes");
Console.ReadLine();
}
}
A class is simply a representation of a type of object. It is the blueprint / plan / template that describes the details of an object. A class is the blueprint from which the individual objects are created.
For example:
Take an example of a bowl in which you can bake a cake, the cake could be of different flavors so cake is my class and its flavors are objects.

CODE:
How can you create a class and object in C#?
class Program{
static void Main(string[] args)
{
baby aisha = new baby();
aisha.crawl();
Console.WriteLine("i am too little so i can crawl only :-)");
Console.ReadLine();
aisha.food();
Console.WriteLine("i eat mashed potatoes");
Console.ReadLine();
}
}
class baby{
public void crawl()
{
public void crawl()
{
}
public void food()
{
public void food()
{
}
}

Why method of main is static?
Static methods can't be accessed by an object, but can be accessed by the class.
Why ReadLine or Readkey?
ReadLine takes the whole line and we can zoom our window by pressing more than one key while ReadKey takes a word and back to the code window.
What is the concept of method?
All processes done by an object could be a method. A method is like a human being, my peon is my method, I called my peon and assigned some task, or remote is my method I do call remote and slow AC.
- Data type of method.
- Access modification.
- Method name.
- Method definition
- Method declaration
- Method call.
- Parameters.
- Method signature.
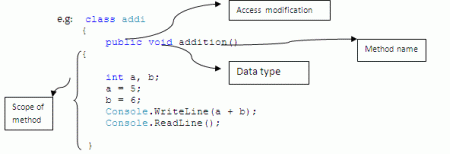
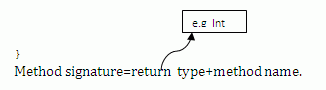
C# sum two numbers:
class Program{
static void Main(string[] args)
{
addi stat = new addi();
stat.addition();
Console.ReadLine();
}
}
class Program{
static void Main(string[] args)
{
addi stat = new addi();
stat.addition();
Console.ReadLine();
}
}
Output: 11
What is Method overload?
Within the same class more than one method can exist but with different parameters.
Code:
class Program{
static void Main(string[] args)
{
won stat = new won();
stat.Add(4,2,1,2);
Console.WriteLine(stat.Add(4,2,1,2));
Console.ReadLine();
static void Main(string[] args)
{
addi stat = new addi();
stat.addition();
Console.ReadLine();
}
}
Output: 11
What is Method overload?
Within the same class more than one method can exist but with different parameters.
Code:
class Program{
static void Main(string[] args)
{
won stat = new won();
stat.Add(4,2,1,2);
Console.WriteLine(stat.Add(4,2,1,2));
Console.ReadLine();
}
}
class won{
public int Add(int a,int b)
}
class won{
public int Add(int a,int b)
{return a+b;}
public int Add(int a,int b,int c)
{return a+b+c;}
public int Add(int a,int b,int c,int d)
{return a+b+c+d;}
public int Add(int a,int b,int c,int d)
{return a+b+c+d;}
}
So what did you learn from overloading?
- There could be two or more methods with the same name.
- But with a different number of parameters of the same data type.
- Or with a different data type of the same number of parameters.
- There could be two or more methods with a different signature.
- When the number of parameters is the same then the data type of the parameter is different or vice versa.
"In two different classes there could be methods with same name."
class Program{
static void Main(string[] args)
{
class Program{
static void Main(string[] args)
{
fa stats = new fa();
stats.addition(1, 2);
Console.WriteLine(stats.addition(1, 2));
am probability = new am();
probability.addition(2, 3);
Console.WriteLine(probability.addition(2, 3));
Console.ReadLine();
}
}
stats.addition(1, 2);
Console.WriteLine(stats.addition(1, 2));
am probability = new am();
probability.addition(2, 3);
Console.WriteLine(probability.addition(2, 3));
Console.ReadLine();
}
}
class fa{
public int addition(int a, int b)
{
return a + b;
public int addition(int a, int b)
{
return a + b;
}
}class am{
public int addition(int a, int b)
{
return a + b;
}
}
What is Method Overriding?
Method overriding is a language feature that allows a subclass to override a specific implementation of a method that is already provided by one of its super-classes.
A subclass can give its own definition of methods but needs to have the same signature as the method in its super-class. This means that when overriding a method the subclass's method has to have the same name and parameter list as the super-class's overridden method.
What is Abstraction?
Sometimes there is a class which does not create objects; this class provides a definition to other classes and this is known as an abstraction.
For example:
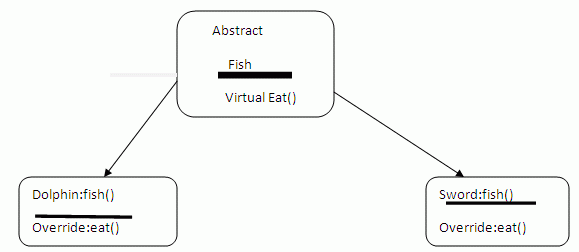
Consider fish is a class. In class fish there are two types of fish dolphin and sword; both have different food. An abstract class specifies that if a fish is a parent class and when the concept of inheritance is implemented;
fish says eat, after being inherited then the Dolphin says eat vegetables now I want to override the child method so we made the parent class virtual.
What is Polymorphism?
Polymorphism comes with overriding. To provide many ways to do the single thing.
Polymorphisms means the ability to request that the same operations be performed by a wide range of different types of things.
What are namespaces?
Namespace is a wrapper which groups classes together, namespace within namespace can be exist.
Interface: C# can't perform multiple inheritance so we adopt the term "interface". An interface is just like a class; a class is a reference type while an interface is a value type.
An interface looks like a class, but has no implementation. An interface is equal to an abstract class but an abstract class can't perform multiple inheritances that an interface can perform.
C# code:
interface IParentInterface{
void ParentInterfaceMethod();
}
}class am{
public int addition(int a, int b)
{
return a + b;
}
}
What is Method Overriding?
Method overriding is a language feature that allows a subclass to override a specific implementation of a method that is already provided by one of its super-classes.
A subclass can give its own definition of methods but needs to have the same signature as the method in its super-class. This means that when overriding a method the subclass's method has to have the same name and parameter list as the super-class's overridden method.
What is Abstraction?
Sometimes there is a class which does not create objects; this class provides a definition to other classes and this is known as an abstraction.
For example:
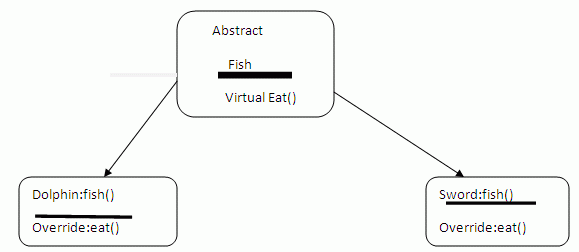
Consider fish is a class. In class fish there are two types of fish dolphin and sword; both have different food. An abstract class specifies that if a fish is a parent class and when the concept of inheritance is implemented;
fish says eat, after being inherited then the Dolphin says eat vegetables now I want to override the child method so we made the parent class virtual.
What is Polymorphism?
Polymorphism comes with overriding. To provide many ways to do the single thing.
Polymorphisms means the ability to request that the same operations be performed by a wide range of different types of things.
What are namespaces?
Namespace is a wrapper which groups classes together, namespace within namespace can be exist.
Interface: C# can't perform multiple inheritance so we adopt the term "interface". An interface is just like a class; a class is a reference type while an interface is a value type.
An interface looks like a class, but has no implementation. An interface is equal to an abstract class but an abstract class can't perform multiple inheritances that an interface can perform.
C# code:
interface IParentInterface{
void ParentInterfaceMethod();
}
interface IMyInterface : IParentInterface{
void MethodToImplement();
}
void MethodToImplement();
}
class InterfaceImplementer : IMyInterface{
static void Main()
{
InterfaceImplementer iImp = new InterfaceImplementer();
iImp.MethodToImplement();
iImp.ParentInterfaceMethod();
}
static void Main()
{
InterfaceImplementer iImp = new InterfaceImplementer();
iImp.MethodToImplement();
iImp.ParentInterfaceMethod();
}
public void MethodToImplement()
{
Console.WriteLine("MethodToImplement() called.");
}
public void ParentInterfaceMethod()
{
Console.WriteLine("ParentInterfaceMethod() called.");
}
}
Loops
In C# there are four loops:
{
Console.WriteLine("MethodToImplement() called.");
}
public void ParentInterfaceMethod()
{
Console.WriteLine("ParentInterfaceMethod() called.");
}
}
Loops
In C# there are four loops:
- For
- While
- Do while
- Foreach
1. The For loop:
It's preferred when you know how many iterations you want, either because you know the exact amount of iterations, or because you have a variable containing the amount.
using System;
namespace ConsoleApplication1
{
class Program {
static void Main(string[] args)
{
int number = 5;
It's preferred when you know how many iterations you want, either because you know the exact amount of iterations, or because you have a variable containing the amount.
using System;
namespace ConsoleApplication1
{
class Program {
static void Main(string[] args)
{
int number = 5;
for (int i = 0; i < number; i++)
Console.WriteLine(i);
Console.ReadLine();
}
}
}
2.The While loop
The while loop simply executes a block of code as long as the condition you give it is true.
namespace ConsoleApplication1
{
class Program {
static void Main(string[] args)
{
int number = 0;
Console.WriteLine(i);
Console.ReadLine();
}
}
}
2.The While loop
The while loop simply executes a block of code as long as the condition you give it is true.
namespace ConsoleApplication1
{
class Program {
static void Main(string[] args)
{
int number = 0;
while (number < 5)
{
Console.WriteLine(number);
number = number + 1;
}
{
Console.WriteLine(number);
number = number + 1;
}
Console.ReadLine();
}
}
}
3. The do loop
do{
Console.WriteLine(number);
number = number + 1;
} while (number < 5);
A do while loop is helpful for viruses and for security level checking.
4. The foreach loop
A foreach loop operates on collections of items, for instance arrays or other built-in list types.
using System;using System.Collections;
}
}
}
3. The do loop
do{
Console.WriteLine(number);
number = number + 1;
} while (number < 5);
A do while loop is helpful for viruses and for security level checking.
4. The foreach loop
A foreach loop operates on collections of items, for instance arrays or other built-in list types.
using System;using System.Collections;
namespace ConsoleApplication1
{
class Program {
static void Main(string[] args)
{
ArrayList list = new ArrayList();
list.Add("cat");
list.Add("cow");
list.Add("fish");
{
class Program {
static void Main(string[] args)
{
ArrayList list = new ArrayList();
list.Add("cat");
list.Add("cow");
list.Add("fish");
foreach (string name in list)
Console.WriteLine(name);
Console.ReadLine();
}
}
}
Switch case
The switch statement is a control statement that handles multiple selections by passing control to one of the case statements within its body.
namespace ConsoleApplication9
{
class Program {
static void Main(string[] args)
{
int caseSwitch = 1;
switch (caseSwitch)
{
case 1:
Console.WriteLine("hello");
break;
case 2:
Console.WriteLine("hi");
break;
default:
Console.WriteLine("Default case");
break;
Console.WriteLine(name);
Console.ReadLine();
}
}
}
Switch case
The switch statement is a control statement that handles multiple selections by passing control to one of the case statements within its body.
namespace ConsoleApplication9
{
class Program {
static void Main(string[] args)
{
int caseSwitch = 1;
switch (caseSwitch)
{
case 1:
Console.WriteLine("hello");
break;
case 2:
Console.WriteLine("hi");
break;
default:
Console.WriteLine("Default case");
break;
}
}
}
}
}
}